props
props简单来说就是向组件中传递数据。很多时候,我们都有类似的需求:我们正在构建一个博客,我们可能需要一个表示博客文章的组件。我们希望所有的博客文章分享相同的视觉布局,但有不同的内容。要实现这样的效果自然必须向组件中传递数据,例如每篇文章标题和内容,这就会使用到 props。
假如我们需要传递给我们博客文章一个标题,我们需要在组件template
同级的属性props
中声明
1 2 3 4 5 6 7 8
| export default { template: ` <h4> {{title}} </h4> `, props: { title: String } }
|
然后我们可以在父组件这样写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| import Test from "./Test.js";
export default { components: {Test}, template: ` <test :title="title1"></test> <test :title="title2"></test> `, data() { return { title1: 'test1', title2: 'test2', } } }
|
这样就可以从父组件传递值给Test组件,最终效果如下
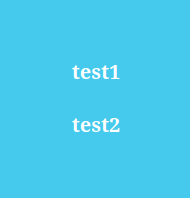
结合蛋老师的视频例子来看,我们发现已购清单
和未购清单
的结构是相同的,因此我们可以写成一个组件,并且利用props达到组件复用的效果
我们可以新建一个AppSectionsList.js
来编写我们的子组件,将我们改写后的组件放入
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| export default { template: ` <section v-show="filter.length"> <h2>{{ title }}</h2> <ul> <li v-for="food in filter" :key="food.id"> <img :src="food.image"> <span>{{ food.name }}</span> <input type="checkbox" v-model="food.purchased"> </li> </ul> </section> `, props: { title: String, filter: Array } }
|
然后我们要修改我们的父组件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| import AppSectionsList from "./AppSectionsList.js";
export default { components: {AppSectionsList}, template: ` <app-sections-list title="已购清单" :filter="filters.afterBuy"> </app-sections-list> <app-sections-list title="未购清单" :filter="filters.beforeBuy"> </app-sections-list> `, data() { return { foods: [ { id: 1, name: '原味鱿鱼丝', image: './images/原味鱿鱼丝.png', purchased: false }, { id: 2, name: '辣味鱿鱼丝', image: './images/辣味鱿鱼丝.png', purchased: false }, { id: 3, name: '炭烧味鱿鱼丝', image: './images/炭烧味鱿鱼丝.png', purchased: false } ] } }, computed: { filters() { return { beforeBuy: this.foods.filter(item => !item.purchased), afterBuy: this.foods.filter(item => item.purchased) } } } }
|